Manage using API¶
API keys can be programmatically managed. Prerequisite is to have an API key with permissions allowing API key module operations. Dashboard and API key wizard can be used to quickly create such API key as explained in Manage using dashboard. Such API key might have following permissions:
Path | Methods | ACL |
---|---|---|
/api-keys/core\.api-key/[0-9a-fA-F]{24} | POST, PATCH, DELETE | off |
/api-keys/core\.api-key | GET, POST | off |
Create¶
Example of an API request creating an API key:
curl -H 'Content-Type: application/vnd.api+json' \
-H 'Accept: application/vnd.api+json' \
-H 'api-key: <YOUR_API_KEY_SECURE_ID>' \
-H 'application-id: <YOUR_APPLICATION_ID>' \
-d '
{
"data": {
"type": "core.api-key",
"attributes": {
"name": "Programmatic API key",
"permissions": [
{
"path": "/resources/my\\.type/[0-9a-fA-F]{24}",
"methods": [ "get" ]
},
{
"path": "/resources/my\\.type/[0-9a-fA-F]{24}",
"methods": [ "patch" ],
"acl": {
"active": true
}
}
]
}
}
}' \
-X POST https://api.jazer.io/api-keys/core.api-key
API key data must contain attributes:
- name - API key name
- permissions - an array of permission objects
Permission object must contain properties:
- path - permission path expressed as a regular expression
- methods - an array of strings get, post, patch and delete
- acl - (optional) object defining ACL
ACL object can contain properties:
- active - boolean, when true permission undergoes ACL
- endpoint-access-list-flavor - string enum with values black and white. Controls the endpoint-access-list to act as a black or a white list. When black all users will have access to the endpoint except the ones covered by the list. When white only users covered by the list will have access to the endpoint and others will not. Default is black.
- endpoint-access-list - array of objects containing properties type and id, which are authentication identities (users and user groups)
- document-access-list-flavor - string enum with values black and white. Controls the document-access-list to act as a black or a white list. When black only users covered by the list will undergo document ACL and for the others document ACL will not be active. When white all users will undergo document ACL except the ones covered by the list. Default is white.
- document-access-list - array of objects containing properties type and id, which are authentication identities (users and user groups)
The system will create API key with a unique ID and secure ID. API will return a response like:
{
"data": {
"type": "core.api-key",
"id": "5c4b18bea7d387048c8e9729",
"attributes": {
"secure-id": "$2a$11$NZdrLF2yQQTtr4odOBDQY.64cIvY2SatlLOKsEor4PZzEwx3aYRpq",
"name": "Programmatic API key",
"permissions": [
{
"path": "/resources/my\\.type/[0-9a-fA-F]{24}",
"methods": [
"get"
]
},
{
"path": "/resources/my\\.type/[0-9a-fA-F]{24}",
"methods": [
"patch"
],
"acl": {
"active": true
}
}
]
},
"links": {
"self": "https://api.jazer.io/api-keys/core.api-key/5c4b18bea7d387048c8e9729"
}
}
}
The same API key can be viewed on the dashboard:
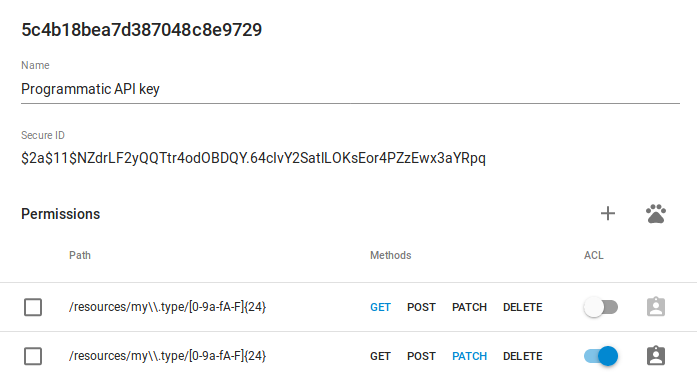
Read¶
Fetching API key by ID can be achieved using following API request:
curl -H 'Accept: application/vnd.api+json' \
-H 'api-key: <YOUR_API_KEY_SECURE_ID>' \
-H 'application-id: <YOUR_APPLICATION_ID>' \
-X GET https://api.jazer.io/api-keys/core.api-key/<API_KEY_ID>
Update¶
To change permissions of an existing API key, following API request can be used:
curl -H 'Content-Type: application/vnd.api+json' \
-H 'Accept: application/vnd.api+json' \
-H 'api-key: <YOUR_API_KEY_SECURE_ID>' \
-H 'application-id: <YOUR_APPLICATION_ID>' \
-d '
{
"data": {
"type": "core.api-key",
"id": "<API_KEY_ID>",
"attributes": {
"permissions": [
{
"path": "/resources/my\\\\.type/[0-9a-fA-F]{24}",
"methods": [ "get" ]
}
]
}
}
}' \
-X PATCH https://api.jazer.io/api-keys/core.api-key/<API_KEY_ID>
Delete¶
To remove API key use following API request:
curl -H 'Accept: application/vnd.api+json' \
-H 'api-key: <YOUR_API_KEY_SECURE_ID>' \
-H 'application-id: <YOUR_APPLICATION_ID>' \
-X DELETE https://api.jazer.io/api-keys/core.api-key/<API_KEY_ID>
Search¶
Search is enabled on API keys endpoint. API request to fetch API keys is:
curl -H 'Accept: application/vnd.api+json' \
-H 'api-key: <YOUR_API_KEY_SECURE_ID>' \
-H 'application-id: <YOUR_APPLICATION_ID>' \
-X GET https://api.jazer.io/api-keys/core.api-key
Search features like filtering, sorting, pagination etc. are available as in Search in resources module.